To use props in React, you need to follow these steps:
1. Define a component that accepts props: Start by creating a component that expects to receive props as input. This can be either a functional component or a class component.
For functional components:
import React from 'react';
const MyComponent = (props) => {
// Component logic using props
return <div>{props.message}</div>;
};
For class components:
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
// Component logic using this.props
return <div>{this.props.message}</div>;
}
}
2. Pass props to the component: In a parent component or the component that renders the component with props, you can pass the props by adding attributes to the JSX tag.
// Parent component or component that renders MyComponent
<MyComponent message="Hello, World!" />
3. Access props within the component: In the component receiving the props, you can access the values through the `props` object. For functional components, use `props.propName`, and for class components, use `this.props.propName`.
// Functional component
const MyComponent = (props) => {
return <div>{props.message}</div>;
};
// Class component
class MyComponent extends Component {
render() {
return <div>{this.props.message}</div>;
}
}
In the example above, the prop `message` is passed as an attribute when rendering the `MyComponent`. Within the component, the prop value is accessed using `props.message` for functional components and `this.props.message` for class components.
Props can be used to pass any type of data, including strings, numbers, objects, arrays, or even functions, from parent components to child components. The child components can then use these props to render dynamic content or perform certain actions based on the provided data.
Remember to ensure that the prop names are consistent between the parent component and the child component to avoid any issues when accessing the props.
Other Example
Create a Menu.js
import './App.css'
function Menu(props){
// Component logic using props
return <div className='box'>{props.message}</div>;
};
export default Menu;
./App.css
.box{
width: 25%;
height: auto;
background-color: aqua;
border: solid 5 px black;
border-radius: 20%;
margin: auto;
text-align: center;
font-size: large;
padding: 10px;
margin-top: 10px;
}
./ App.js
import './App.css';
//@p4n.in
import Menu from './Menu';
function App() {
let course= ['java','c++','jsp','python','go','r']
let size= course.length;
return(
<>
{[...Array(size)].map((x,i) =>
<Menu message={course[i]} />
)}
</>
);
}
export default App;
output :
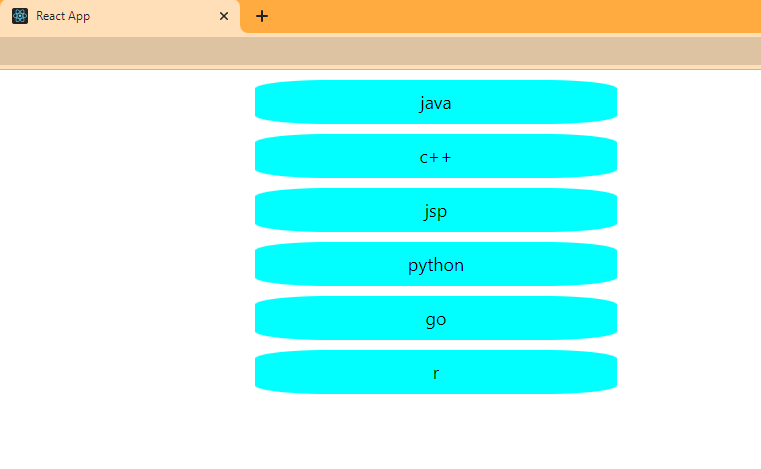
Komentarze