Forms are really important in any website for login, signup, or whatever. It is easy to make a form in HTML but forms in React work a little differently. In HTML the form data is usually handled by the DOM itself but in the case of react the form data is handled by the react components. All the form data is stored in the react’s component state, so it can handle the form submission and retrieve data that the user entered. To do this we use controlled components.
Handling Forms
In React all the data is handled by component and stored in component state. We can change state with event handlers in the onChange attribute, like this:
import { useState } from 'react';
function Form() {
const [email, setEmail] = useState('');
return (
<form>
<label>
Enter your email: <input type="email" value={email} onChange={(e) => setEmail(e.target.value)} />
</label>
</form>
)
}
export default Form;
Submitting Form
We can submit form with onSubmit attribute for the <form>
import { useState } from 'react';
function Form() {
const [email, setEmail] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
alert(`Your email is: ${email}`)
}
return (
<form onSubmit={handleSubmit}>
<label>
Enter your email: <input type="email" value={email} onChange={(e) => setEmail(e.target.value)} />
</label>
<input type="submit" />
</form>
)
}
export default Form;
Multiple Inputs
We don't have to make multiple states for multiple inputs, we can save all values in one, like this:
import { useState } from 'react';
function Form() {
const [data, setData] = useState({});
const handleChange = (e)=>{
setData({...data, [e.target.name]: e.target.value})
}
const handleSubmit = (e) => {
e.preventDefault();
alert(`Your email is: ${data.email} and your name is: ${data.name}`)
}
return (
<form onSubmit={handleSubmit}>
<label>
Enter your email: <input type="email" name='email' value={data.email} onChange={handleChange} />
</label>
<label>
Enter your name: <input type="text" name='name' value={data.name} onChange={handleChange} />
</label>
<input type="submit" />
</form>
)
}
export default Form;
Here in handleChange we used Spread Operators. We are basically saying keep whole data as it was, just change this name's value. Then it is saved as an object so we are getting that by data.email and data.name.
Example 1: In this example code, we are going to console log the value of the input field with the help of the onInputChange function. and we’ll see that every time the user input we get the output on the console of the browser.
First, create react app, and from your project directory update your index.js file from the src folder.
src index.js:
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
onInputChange(event) {
console.log(event.target.value);
}
render() {
return (
<div>
<form>
<label>Enter text</label>
<input type="text"
onChange={this.onInputChange}/>
</form>
</div>
);
}
}
ReactDOM.render(<App />,
document.querySelector('#root'));
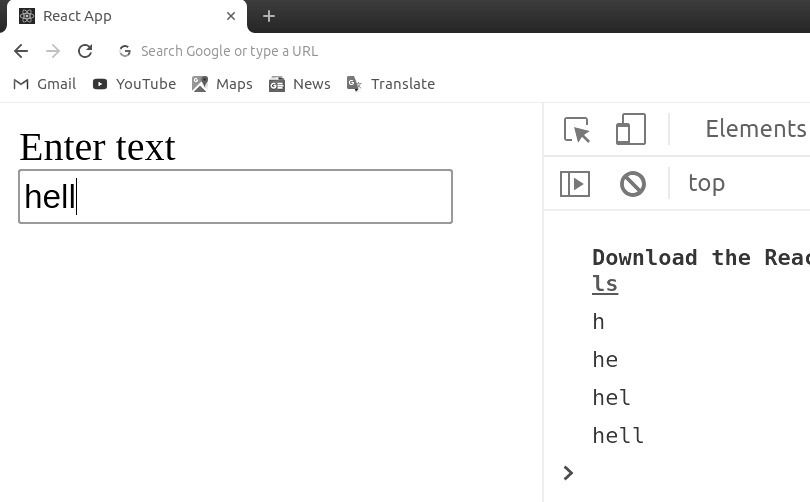
Example 2: Now let’s, again change the src/index.js with the below-given code, and in this code, we are updating the value of inputValue each time user changes the value in the input field by calling the setState() function.
Src index.js:
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
state = { inputValue: '' };
render() {
return (
<div>
<form>
<label> Enter text </label>
<input type="text"
value={this.state.inputValue}
onChange={(e) => this.setState(
{ inputValue: e.target.value })} />
</form>
<br />
<div>
Entered Value: {this.state.inputValue}
</div>
</div>
);
}
}
ReactDOM.render(<App />,
document.querySelector('#root'));
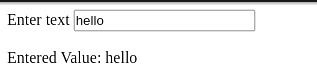
Example 3: Here we just added the onSubmit event handler which calls the function onFormSubmit and it prevents the browser from submitting the form and reloading the page and changing the input and output value to ‘Hello World!’.
src index.js:
import React from 'react';
import ReactDOM from 'react-dom';
class App extends React.Component {
state = { inputValue: '' };
onFormSubmit = (event) => {
event.preventDefault();
this.setState({ inputValue: 'Codeswithpankaj' });
}
render() {
return (
<div>
<form onSubmit={this.onFormSubmit}>
<label> Enter text </label>
<input type="text"
value={this.state.inputValue}
onChange={(e) => this.setState(
{ inputValue: e.target.value })} />
</form>
<br />
<div>
Entered Value: {this.state.inputValue}
</div>
</div>
);
}
}
ReactDOM.render(<App />,
document.querySelector('#root'));
Comentarios